The Leap Motion Java SDK uses a standard Jar file for Leap Motion API class definitions and a set of native libraries that allow your Leap-enabled Java programs to exchange data with the Leap. Setting up a Java project typically involves adding the LeapJava.jar file to your application’s classpath and setting the JVM library path parameter so that your JVM can find the native libraries.
Leap Motion Java libraries¶
The Leap Motion Jar file is cross-platform, but the native libraries must match the platform and architecture of the JVM used to run the program.
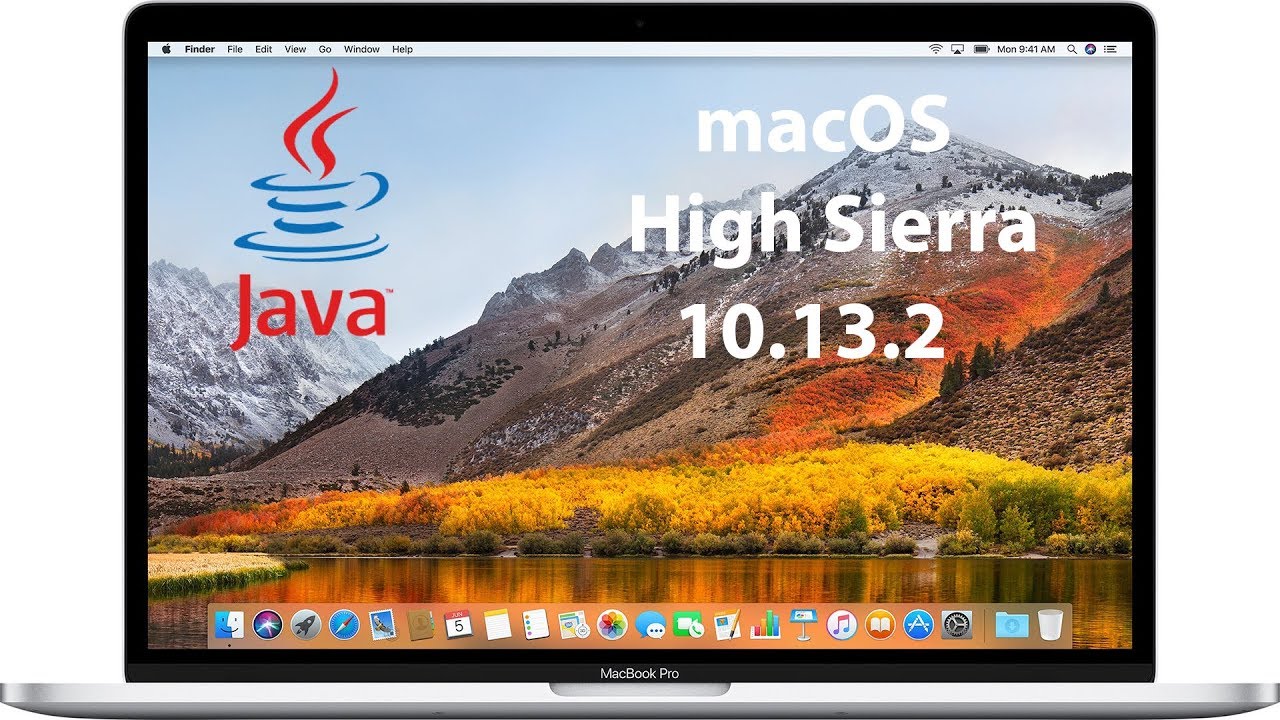
To use the Leap Motion SDK in a Java program, you must add the LeapJava.jar file to the classpath and set the java.library.path to the location of the Leap Motion native libraries.
Use the following Java and native libraries with the Leap Motion Java SDK:
- 32-bit Windows:
- LeapSDK/lib/LeapJava.jar — Leap Motion Java API class definitions
- LeapSDK/lib/x86/LeapJava.dll — 32-bit Leap Motion Java library for Windows
- LeapSDK/lib/x86/Leap.dll — 32-bit Leap Motion library for Windows
- 64-bit Windows:
- LeapSDK/lib/LeapJava.jar — Leap Motion Java API class definitions
- LeapSDK/lib/x64/LeapJava.dll — 64-bit Leap Motion Java library for Windows
- LeapSDK/lib/x64/Leap.dll — 64-bit Leap Motion library for Windows
- 32- or 64-bit Mac OS:
- LeapSDK/lib/LeapJava.jar — Leap Motion Java API class definitions
- LeapSDK/lib/libLeapJava.dylib — Leap Motion Java library for Mac
- LeapSDK/lib/libLeap.dylib — Leap Motion library for Mac
This will unpack and launch the installer program for the Java 2 SDK. Follow the steps on the installer and from the page listed in step 9 to complete the install. Be particularly careful when modifying your autoexec.bat file. Follow the steps on the Java instruction page very closely. Now you should have the Java SDK installed. Java runtime 1.3.1 comes bundled with the OS. JDK up to 1.4.1 and development tools are free downloads/updates from Apple (and I think the recent software update brings 1.4.1 to the masses). Go to apple.com and choose the 'Developer' tab for juicy details. Install java 1.5 sdk on Mac OS 10.7? Ask Question Asked 9 years, 3 months ago. Active 7 years, 11 months ago. Viewed 6k times 3. Mac OS 10.7 (Lion) comes with JDK. Java package structure of Mac OS is a bit different from Windows. Don't be upset for this as a developer just needs to set PATH and JAVAHOME. So in.bashprofile set JAVAHOME and PATH as below.
Compile from the command line¶
Use the Java compiler, javac to compile, setting the classpath option to specify the LeapJar file. For example, to compile the Sample.java program included in the Leap Motion SDK, you could use the following command:
Install Java SDK on MacOS You need to install Java SDK on MacOS to allow a lot of applications and development tools to run in your machine. This post describes the most important installation steps to get a working Java development environment. Download the Java SDK Package.
(where <LeapSDK> is the location of your Leap Motion SDK folder.)

Run from the command line¶
To launch a Leap-enabled program, Java needs to find the Leap Motion native libraries at runtime. LeapJava.jar must also be on the classpath. You can set Java’s java.library.path parameter to identify the native library. The command line syntax is slightly different between Mac and Windows. More importantly, on Windows, you have to specify either the 32-bit or the 64-bit libraries to match the architecture of the JVM you are using.
On Mac, you could run the Sample program using the following command:
On Windows, you could run the Sample program using a 64-bit JVM with the following command:
Eclipse¶
In the Eclipse IDE, you add the LeapJava.jar file to a project as an external Jar and then set the path to the appropriate native Leap Motion libraries as a property of the Jar file.
- Select New > Java Project from the Eclipse File menu.
- Assign a name to the project on the Create Java Project page and set other properties as desired. (The Leap Motion SDK supports Java 6 and 7.)
- Click Next to advance to the Java Settings page.
- Select the Libraries tab.
- Click the Add External Jars... button.
- Navigate to the LeapJava.jar file.
- Click Open to add LeapJava.jar to the project.
- Next, click the small triangle in front of the LeapJava.jar entry in the library list to reveal the library properties.
- Select the Native library location item.
- Click the Edit button.
- Navigate to the folder containing the Leap Motion native libraries.
- Click Ok to set the path.
Note: you can also add the Leap Motion libraries to an existing project from the Project Properties dialog.
IntelliJ¶
In the IntelliJ IDE, you add the LeapJava.jar file to a project as a library. You separately set the path to the Leap Motion native libraries using the JVM parameter, java.library.path. The JVM parameters can be set using an IntelliJ Run/Debug configuration.
To add LeapJava.jar to the project:
- After creating a project in the usual way, select the File > Project Structure menu command to open the settings dialog.
- Click Libraries under project settings.
- Click the small + button at the top of the library list to open the Select Library Files dialog.
- Add LeapJava.jar from your Leap Motion SDK.
To set the path to the native Leap Motion libraries by creating a Run/Debug configuration:

- Select the Run > Edit Configurations… menu command.
- Click the small + button above the Configuration list.
- Choose Application to create a new application configuration.
- Assign a name.
- Set the VM Options field to set the -Djava.library.path parameter to the path to the proper folder containing the Leap Motion native libraries.
- Click Ok.
On Windows, be sure to select the folder containing the correct libraries for your target architecture. If you are targeting a 32-bit JVM, use the Leap Motion libraries in the x86 folder of the SDK. If you are targeting a 64-bit JVM, use the libraries in the x64 folder. On Mac, each Leap Motion library file supports both architectures.
NetBeans¶
In the NetBeans IDE, you add the LeapJava.jar file to a project as a library. You separately set the path to the Leap Motion native libraries using the JVM parameter, java.library.path. The JVM parameters can be set using a NetBeans Run configuration.
To add the LeapJava.jar to a project and set the path to the native libraries:
- After creating a project in the usual way, select the File > Project Properties menu command to open the Project Properties dialog.
- Click the Libraries item.
- Click Add Jar/Folder button.
- Find the LeapJava.jar file in your Leap Motion SDK.
- Click Ok to add the Jar file to your project.
- Next, click the Run item in the Project properties list
- Set the VM Options field to set the -Djava.library.path parameter to the path to the proper folder containing the Leap Motion native libraries.
On Windows, be sure to select the folder containing the correct libraries for your target architecture. If you are targeting a 32-bit JVM, use the Leap Motion libraries in the x86 folder of the SDK. If you are targeting a 64-bit JVM, use the libraries in the x64 folder. On Mac, each Leap Motion library file supports both architectures.
UT Computer Science Installing Java on your computer. |
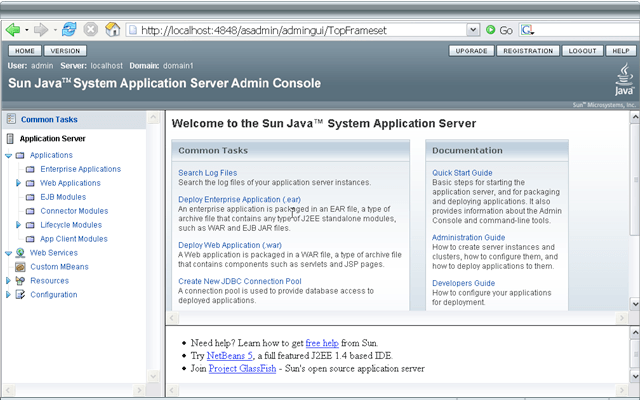
Note: Please remember the teaching staff does not support orassist in setting up software for use at home. If you are having problemsyou should post a question to the class discussion group so others can offer advice or tell you what they did. You may email Mike or your TA if you think you have done everything correctly, but he will may not be able to assist you with your specific problem. Working at home has its benefits and I recommend you download the Java Platform Standard Edition and either Eclipse or BlueJ if you have a computer at home.
Please go tohere to download the Download Java Standard Edition 8.0 Development Kit. You want the JDK. Not just the JRE or Server JRE.
Java 2 Sdk Mac Os
The installation instructions for Windows machines arehere.Instructions for Linux arehere. For Mac's go to this page.
Java 2 Sdk Mac Download
Pick the appropriate link. I will talk through what to do if you are using Windows. If you are using a Macintosh clickhere for a page with instructions on how to download and install Java for the Mac. Ifyou are using Linux I assume you don't need much help and you can just click onthe Linux download and follow the instructions from their.
To download and install the Java Platform Standard Edition Development Kit.
- From the page http://www.oracle.com/technetwork/java/javase/downloads/index.html click on the 'Download JDK' button in the Java SE 8u101 / 8u102 table near the top. (Note, the update number may be something different than 101/102. Don't worry, about that number. You do not need JavaFX, Java EE, or Netbeans (unless you want to use that IDE.)
- Select your platform. (Weird fact: The License Agreement includes Section Restrictions 3 which states 'You acknowledge that Software is not designed, licensed or intended for use in the design, construction, operation or maintenance of any nuclear facility. ' Earlier versions of Java include restrictions on using Java for software intended 'for use in on-line control of aircraft, air traffic, aircraft navigation or aircraft communications.')
- After downloading click the file icon to start the installer if it does not start automatically.
- After downloading and installing Java I recommend also downloading and installing Eclipse, a powerful, but complex IDE or BlueJ a simple, less powerful IDE. You are free to use whatever IDE (JCreator, BlueJ, DrJava, Emacs, VI, Notepad and command line) you want, but I have support pages for Eclipse and BlueJ.
- If you want to download the Java documentation. Go to the download page where you got the JDK. Expand the 'Additional Resources' menu:
Scroll down until you see the choice for 'Java SE 8 Documentation' and follow the instructions to download the documentation. The process is similar to the JDK, except you will download a zipped file and have to expand it. If you do not have a program on your computer to zip and unzip files (compress and decompress) try 7ZIP which can be downloaded for free. I find it best to bookmark the index page (the local copy on your computer) from the API folder in my web browser.
That's all. If you want more information on Java, there is an excellent tutorial at Sun's Java site.